I had earlier discussed the importance of keeping the android
Every asset we include in the
There are many free icon fonts available online like font-awesome.
We can create custom class for font images by extending Textview
Step by step guide to add font image
- Download icon font to your asset folder
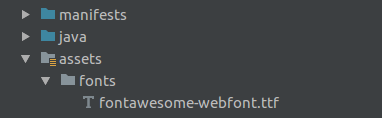
- Use custom class for fonts that extends Textview
public class TextViewAwesomeFont extends TextView {
...
}
- Create Typeface for font file available in asset folder
Typeface face = Typeface.createFromAsset(context.getAssets(), "fonts/fontawesome-webfont.ttf");
this.setTypeface(face);
- Set text size relative to layout width and height
getViewTreeObserver().addOnGlobalLayoutListener(
new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
int width = tv.getWidth();
int height = tv.getHeight();
tv.setTextSize(TypedValue.COMPLEX_UNIT_PX, Math.min(width, height) * 1f);
}
});
- XML layout
<com.example.view.VtnTextViewAwesomeFont
android:layout_width="96dp"
android:layout_height="96dp"
android:textColor="#FFFFFF"
android:text="@string/fa_offline"
/>
- Strings declaration for icons
<string name="fa_offline"></string>
<string name="fa_noresults"></string>
Example for custom class
public class TextViewAwesomeFont extends TextView {
private TextView tv;
public TextViewAwesomeFont(Context context) {
super(context);
RenderIcon(context);
}
public TextViewAwesomeFont(Context context, AttributeSet attrs) {
super(context, attrs);
RenderIcon(context);
}
public TextViewAwesomeFont(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
RenderIcon(context);
}
@TargetApi(Build.VERSION_CODES.LOLLIPOP)
public TextViewAwesomeFont(Context context, AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
RenderIcon(context);
}
private void RenderIcon(Context context)
{
tv = this;
Typeface face = Typeface.createFromAsset(context.getAssets(), "fonts/fontawesome-webfont.ttf");
this.setTypeface(face);
this.setSingleLine();
getViewTreeObserver().addOnGlobalLayoutListener(
new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
int width = tv.getWidth();
int height = tv.getHeight();
tv.setTextSize(TypedValue.COMPLEX_UNIT_PX, Math.min(width, height) * 1f);
}
});
}
}
What are your thoughts on this article? reach out to us at info@ventunotech.com if you would like to discuss!
Looking to launch your own streaming service?
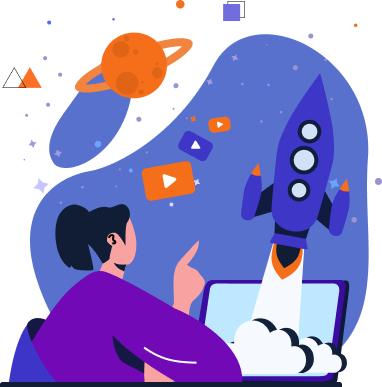